This subchapter is provided as a free sample for SwiftUI Fundamentals book.
You can read this subchapter online or download the sample bundle with PDF and EPUB by clicking the link below.
Download free sampleTo get access to the contents of the whole book you need to purchase a copy.
FREE SAMPLE - online version
Styling Text views
SwiftUI provides several approaches to styling text, allowing us to create visually rich and dynamic content. By leveraging view modifiers, text-specific modifiers, Markdown, or attributed strings, we can tailor text to fit a variety of design requirements.
# View modifiers
We can style text in SwiftUI using view modifiers, such as font(_:)
, foregroundStyle(_:)
, and many others. These modifiers can be applied directly to a Text
view to customize its appearance or to a container view to apply styling across multiple text elements.
Since string labels in SwiftUI controls, such as buttons, are internally represented as Text
, these modifiers also apply to them. This approach simplifies styling and maintains visual consistency within the interface.
VStack {
Text("Now Showing: Inside Out 2")
Button("Get Tickets", action: purchaseTickets)
.buttonStyle(.bordered)
}
.font(.headline)
.fontDesign(.rounded)
Here, both the standalone Text
and the button label adopt the headline font with a rounded design, creating a unified appearance for all text elements in the container.
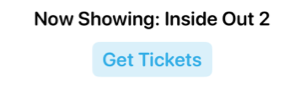
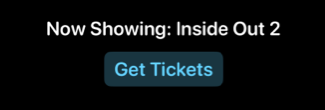
# Text modifiers
In addition to standard view modifiers, which can be applied anywhere in the view hierarchy, SwiftUI provides text-specific modifiers tailored for styling individual Text
views. Unlike view modifiers, which apply to any SwiftUI view and return some View
, text modifiers return another Text
instance when applied directly to Text
. This distinction allows text modifiers to target specific parts of a string, making them particularly effective when working with interpolated or concatenated text.
Text modifiers are ideal for emphasizing particular words or phrases within a sentence. By using interpolation, we can apply styles to individual segments while leaving the surrounding text unchanged.
Text("""
Showtimes: \(Text("Friday").bold()) \
and \(Text("Saturday").bold())
""")
In this example, "Friday" and "Saturday" are styled in bold, visually distinguishing them from the rest of the text, which retains the default font weight.


Modifiers like foregroundStyle(_:)
can also be applied inline to add custom styles, such as colors or gradients.
Text("""
Only \(
Text("25")
.foregroundStyle(
.linearGradient(
colors: [.pink, .purple],
startPoint: .leading,
endPoint: .trailing
)
)
) tickets left!
""")
Here, the number 25
is displayed in a pink and purple gradient, drawing attention to the numeric value while keeping the rest of the text un-styled.


Text interpolation combined with text modifiers provides precise control over formatting, enabling selective styling of text content. This flexibility is invaluable when emphasizing specific details and creating visually engaging text layouts.
# Markdown
SwiftUI supports Markdown formatting within text views when Text
is initialized from a string literal. This feature allows for easy application of text styles such as bold, italic, strikethrough, and monospace. By using Markdown, we can directly embed these styles into the text, eliminating the need for additional modifiers and simplifying the styling process.
Here is how we can emphasize a word with italic style:
Text("See *Spider-Man* on the big screen tonight!")
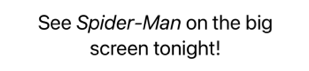
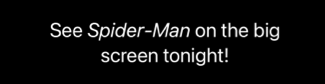
We can also embed links directly in text, and SwiftUI will automatically render them as interactive elements. When a URL is included in a string literal using Markdown syntax, the Text
view detects it and makes it tappable without requiring additional code to handle interactions.
Text("Get tickets on our [website](https://example.com).")


The link adopts the default system styling for interactive text. If necessary, the tint(_:)
modifier can be used to adjust its color.
Text("Get tickets on our [website](https://example.com).")
.tint(.pink)


While SwiftUI supports inline Markdown styles and links, it does not recognize paragraph-level formatting such as headers or code blocks. Markdown in Text
is best suited for simple formatting and inline enhancements.
# Attributed strings
Another way to style portions of text in SwiftUI is by using the AttributedString
APIs. These provide a modern and strictly typed way to apply attributes to text.
We can define an AttributedString
and pass it to a Text
view to style specific parts of the text. For instance, we can set a background color and a foreground color for a substring, and SwiftUI will render it accordingly.
struct PremiereView: View {
var attrString: AttributedString {
var attrString = AttributedString(
"Premiere: The Wild Robot"
)
if let range = attrString.range(
of: "The Wild Robot"
) {
attrString[range].backgroundColor = .mint
attrString[range].foregroundColor = .black
}
return attrString
}
var body: some View {
Text(attrString)
}
}


Attributes for AttributedString
are organized into scopes, with each UI framework, such as UIKit, AppKit, and SwiftUI, defining its own. The SwiftUI scope includes attributes such as foregroundColor
, backgroundColor
, font
, kern
, tracking
, underlineStyle
, strikethroughStyle
, and baselineOffset
. Any attributes not supported by SwiftUI are ignored when a Text
view renders an AttributedString
.
SwiftUI also recognizes some Foundation attributes, such as links and inline presentation intent. Accessibility attributes are included as well, enabling customization for assistive technologies to improve the user experience.